No nonsense temporary file hosting
Serving 0 public files totalling 0kb
Inspired by https://0x0.st, WaifuVault is a temporary file hosting service that allows for file uploads that are hosted for a set amount of time.
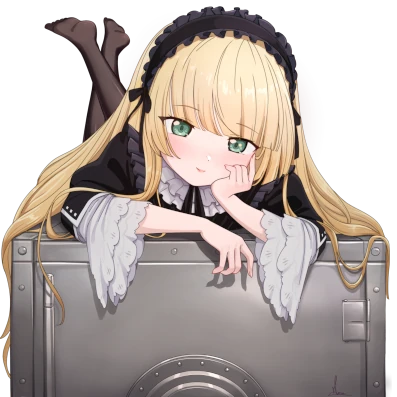
Fast
Through the use of ETags and efficient caching, rapid file access and upload.
Simple
Simple API access for file upload. No authorisation is required and is totally free, just upload your file.
Temporary
All files uploaded are temporary, and will be deleted once their allotted lifespan expires. Shorter lifespans can be specified at upload.
Features
Restrictions
The following cannot be uploaded, and any uploads in violation of this will be banned
- Piracy
- Extremist material
- Malware
- Crypto shit
- Anything illegal under British Law
- File Limit: 100MB
The amount of time a given file is hosted for is determined by its size. Files are hosted for a maximum of 365 days, with the time being shortened on a cubic curve.
This means for files up to about 50% of the maximum file size will get close to the maximum time. All files will be retained for at LEAST 30 days
Use the control below to see how long a file will be retained for
Privacy Policy
No data is shared with third parties, and data will be deleted permanently when the file expires or is deleted by the user
If we believe the release of this information is necessary to respond to a legal process, to investigate or remedy potential violations of our policies, or to protect the rights, property, and safety of others, we may share your information as permitted or required by any applicable law, rule, or regulation.
No uniform technology standard for recognising and implementing Do-Not-Track(DNT) signals has been finalised. As such, we do not currently respond to DNT browser signals or any other mechanism that automatically communicates your choice not to be tracked online.
While we have taken reasonable steps to secure any information you provide to us, please be aware that despite our efforts, no security measures are perfect or impenetrable. No method of data transmission can be guaranteed against any interception or other type of misuse. Any information disclosed online is potentially vulnerable to interception and misuse by unauthorised parties.
We securely hash your IP address using SHA-256.
This hash is only used for bucket validation and is never stored in plain text or logged.
Even we cannot access or determine what your original IP address is.
We also do not request, log or retain any personal information from you.
Bespoke SDKs
Official SDKs for interacting with waifuvault
Examples
Please refer to the Swagger for full api documentation including endpoints and optional arguments you can pass.
// upload file via URL
curl --request PUT --url https://waifuvault.moe/rest --data url=https://victorique.moe/img/slider/Quotes.jpg
// upload file via File
curl --request PUT --url https://waifuvault.moe/rest --header 'Content-Type: multipart/form-data' --form [email protected]
For Node, there is an official SDK that provides access to all of the features of the site.
WaifuVault Node API
import Waifuvault from "waifuvault-node-api";
// upload file
const resp = await Waifuvault.uploadFile({
file: "./files/aCoolFile.jpg"
});
console.log(resp.url); // the file download URL
// upload via URL
const resp = await Waifuvault.uploadFile({
url: "https://waifuvault.moe/assets/custom/images/vic_vault.webp"
});
console.log(resp.url); // the file download URL
// upload a buffer
const resp = await Waifuvault.uploadFile({
file: Buffer.from("someData"),
filename: "aCoolFile.jpg"
});
console.log(resp.url); // the file download URL
For Go, there is an official SDK that provides access to all of the features of the site.
WaifuVault Go API
//upload via URL
package main
import (
"context"
"fmt"
"github.com/waifuvault/waifuVault-go-api/pkg"
waifuMod "github.com/waifuvault/waifuVault-go-api/pkg/mod" // namespace mod
"net/http"
)
func main() {
api := waifuVault.NewWaifuvaltApi(http.Client{})
file, err := api.UploadFile(context.TODO(), waifuMod.WaifuvaultPutOpts{
Url: "https://waifuvault.moe/assets/custom/images/vic_vault.webp",
})
if err != nil {
return
}
fmt.Printf(file.URL) // the URL
}
// upload file
package main
import (
"context"
"fmt"
"github.com/waifuvault/waifuVault-go-api/pkg"
waifuMod "github.com/waifuvault/waifuVault-go-api/pkg/mod"
"net/http"
"os"
)
func main() {
api := waifuVault.NewWaifuvaltApi(http.Client{})
fileStruc, err := os.Open("myCoolFile.jpg")
if err != nil {
fmt.Print(err)
}
file, err := api.UploadFile(context.TODO(), waifuMod.WaifuvaultPutOpts{
File: fileStruc,
})
if err != nil {
return
}
fmt.Printf(file.URL) // the URL
}
// upload buffer
package main
import (
"context"
"fmt"
"github.com/waifuvault/waifuVault-go-api/pkg"
waifuMod "github.com/waifuvault/waifuVault-go-api/pkg/mod"
"net/http"
"os"
)
func main() {
api := waifuVault.NewWaifuvaltApi(http.Client{})
b, err := os.ReadFile("myCoolFile.jpg")
if err != nil {
fmt.Print(err)
}
file, err := api.UploadFile(context.TODO(), waifuMod.WaifuvaultPutOpts{
Bytes: &b,
FileName: "myCoolFile.jpg", // make sure you supply the extension
})
if err != nil {
return
}
fmt.Printf(file.URL) // the URL
}
For Python, there is an official SDK that provides access to all of the features of the site.
WaifuVault Python API
import waifuvault
import io
# upload file
upload_file = waifuvault.FileUpload("./files/aCoolFile.png")
upload_res = waifuvault.upload_file(upload_file)
print(upload_res.url)
# upload via URL
upload_file = waifuvault.FileUpload("https://waifuvault.moe/assets/custom/images/vic_vault.webp")
upload_res = waifuvault.upload_file(upload_file)
print(upload_res.url)
# upload a buffer
with open("./files/aCoolFile.png", "rb") as fh:
buf = io.BytesIO(fh.read())
upload_file = waifuvault.FileUpload(buf, "aCoolFile.png")
upload_res = waifuvault.upload_file(upload_file)
print(upload_res.url)
For C#, there is an official SDK that provides access to all of the features of the site.
WaifuVault C# API
using Waifuvault;
using System.IO;
// Upload file
var uploadFile = new Waifuvault.FileUpload("./aCoolFile.png");
var uploadResp = await Waifuvault.Api.uploadFile(uploadFile);
Console.WriteLine(uploadResp.url);
// Upload via URL
var uploadFile = new Waifuvault.FileUpload("https://waifuvault.moe/assets/custom/images/vic_vault.webp");
var uploadResp = await Waifuvault.Api.uploadFile(uploadFile);
Console.WriteLine(uploadResp.url);
// Upload via Buffer
byte[] buffer = File.ReadAllBytes("./aCoolFile.png");
var uploadFile = new Waifuvault.FileUpload(buffer,"aCoolFile.png");
var uploadResp = await Waifuvault.Api.uploadFile(uploadFile);
Console.WriteLine(uploadResp.url);
For Rust, there is an official SDK that provides access to all of the features of the site.
WaifuVault Rust API
use waifuvault::{
ApiCaller,
api::{WaifuUploadRequest, WaifuResponse}
};
#[tokio::main]
async fn main() -> anyhow::Result<()> {
let caller = ApiCaller::new();
// Upload a file from disk
let request = WaifuUploadRequest::new()
.file("/some/file/path") // Path to a file
.password("set a password") // Set a password
.one_time_download(true); // Delete after first access
let response = caller.upload_file(request).await?;
// Upload a file from a URL
let request = WaifuUploadRequest::new()
.url("https://some-website/image.jpg"); // URL to content
let response = caller.upload_file(request).await?;
// Upload a file from raw bytes
let data = std::fs::read("some/file/path")?;
let request = WaifuUploadRequest::new()
.bytes(data, "name-to-store.rs"); // Raw file content and name to store on the vault
let response = caller.upload_file(request).await?;
Ok(())
}
For PHP, there is an outdated SDK that provides access to managing files only.
WaifuVault PHP API
// Upload file
use ErnestMarcinko\WaifuVault\WaifuApi;
$waifu = new WaifuApi();
$response = $waifu->uploadFile(array(
'file' => __DIR__ . '/image.jpg',
));
var_dump($response);
// Upload via URL
use ErnestMarcinko\WaifuVault\WaifuApi;
$waifu = new WaifuApi();
$response = $waifu->uploadFile(array(
'url' => 'https://waifuvault.moe/assets/custom/images/vic_vault.webp',
));
var_dump($response);
// Upload via Buffer
use ErnestMarcinko\WaifuVault\WaifuApi;
$waifu = new WaifuApi();
$response = $waifu->uploadFile(array(
'file_contents' => file_get_contents(__DIR__ . '/image.jpg'),
'filename' => 'image.jpg',
));
var_dump($response);
// using Apache HttpClient
import org.apache.http.client.ResponseHandler;
import org.apache.http.client.methods.RequestBuilder;
import org.apache.http.entity.mime.HttpMultipartMode;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.nio.file.Path;
public class Example {
private static void uploadFile(final Path file) throws IOException {
try (final var httpclient = HttpClients.createDefault()) {
final var data = MultipartEntityBuilder.create().setMode(HttpMultipartMode.BROWSER_COMPATIBLE)
.addBinaryBody("file", file.toFile())
.build();
final var request = RequestBuilder.put("https://waifuvault.moe/rest").setEntity(data).build();
final ResponseHandler responseHandler = response -> response.getEntity() != null ? EntityUtils.toString(response.getEntity()) : null;
final var responseBody = httpclient.execute(request, responseHandler);
System.out.println(responseBody);
}
}
private static void uploadUrl(final String url) throws IOException {
try (final var httpclient = HttpClients.createDefault()) {
final var data = MultipartEntityBuilder.create().setMode(HttpMultipartMode.BROWSER_COMPATIBLE)
.addTextBody("url", url)
.build();
final var request = RequestBuilder.put("https://waifuvault.moe/rest").setEntity(data).build();
final ResponseHandler responseHandler = response -> response.getEntity() != null ? EntityUtils.toString(response.getEntity()) : null;
final var responseBody = httpclient.execute(request, responseHandler);
System.out.println(responseBody);
}
}
}
For Elixir, there is an official SDK that provides access to all of the features of the site.
WaifuVault Elixir API
require WaifuVault
# Upload file from disk
options = %{}
{:ok, fileResponse} = WaifuVault.upload_local_file("./mix.exs", "my_mix.exs", options)
{:ok, %{...}}
# Upload file from URL
options = %{}
{:ok, fileResponse} = WaifuVault.upload_via_url(image_url, options)
{:ok, %{...}}
# Upload file from buffer
{:ok, buffer} = File.read("some/local/file")
{:ok, fileResponse} = WaifuVault.upload_file_from_buffer(buffer, "file.name", %{expires: "10m"})
{:ok, %{...}}